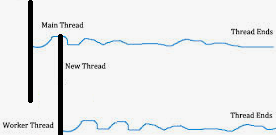
Thread Function, Complete Example
Thread is executed with a function in C#. When the function has exited then the thread has exited too. We shall do an experiment with the following example. In the following windows form application, we have created few buttons and edit boxes for showing the status of the thread. The application has created 3 threads (except the main thread)
private Thread threadOne;
private Thread threadTwo;
private Thread threadObserver;
Observer threads observe the status of the other 2 threads and count the total running threads in the application. In the following snapshot it shows at this moment there are 13 threads (most of the system threads used for this application, we don’t need to care about it). Each thread is blocked by WaitOne method. When we press on the SetOne then we allow the thread to run and do the work. But the thread is running with a function. When the function is set with the Exit flag after that the function will return and the thread status will be Dead. In other case, it will update the what is doing field.
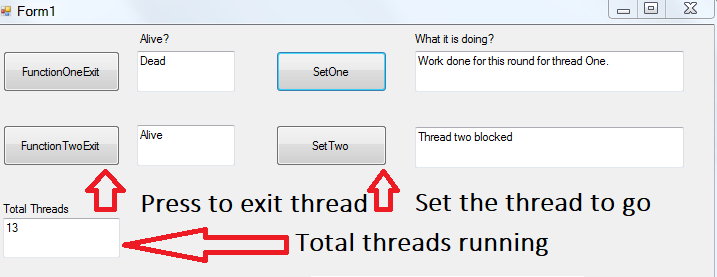
private void WorkMethodOne(object stateInfo)
{
bool b = true;
while (b) //keep the thread alive till b is false
{
lock (lockObject)
{
b = !threadOneExit; // it will be true when the exit button is pressed
}
SetOperationStatusWhatDoesOne("Thread one Blocked");
autoEventOne.WaitOne(); //Block the thread, will start when the Set is pressed
SetOperationStatusWhatDoesOne("Work starting for one.");
Thread.Sleep(5000);
SetOperationStatusWhatDoesOne("Work done for this round for thread One.");
Thread.Sleep(1000);
// ((AutoResetEvent)stateInfo).Set();
}
}
When the exit button is pressed then the flag b will be changed and the function will return and thread will be dead.